カテゴリー【C/C++】
【C++】クラス配列の任意のメンバ変数の総和を求める
POSTED BY
2024-12-15
2024-12-15
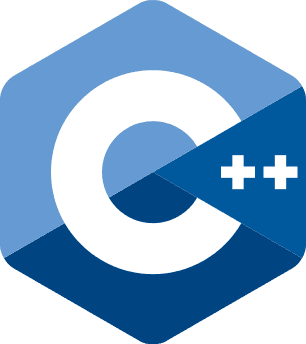
メンバ変数にintとstringを持つ以下のようなクラスがあるとして、
- #include <string>
- class Test {
- public:
- int number;
- std::string string;
- Test(int n, std::string s) {
- number = n;
- string = s;
- }
- };
- </string>
その配列を以下のように確保したとする。
- #include <vector>
- std::vector<test> tests = std::vector<test>();
- tests.push_back(Test(1, std::string("あ")));
- tests.push_back(Test(2, std::string("い")));
- tests.push_back(Test(3, std::string("う")));
- tests.push_back(Test(4, std::string("え")));
- tests.push_back(Test(5, std::string("お")));
- </test></test></vector>
ここで、メンバ変数numberおよびstringの総和を求めるにはstd::accumulateを使う。
- #include <numeric>
- int number_all = std::accumulate( tests.begin(), tests.end(), 0,
- []( int n, auto &c ){ return n + c.number; } );
- std::string string_all = std::accumulate( tests.begin(), tests.end(), std::string(""),
- []( std::string s, auto &c ){ return s + c.string; } );
- </numeric>
第3引数には総和の初期値、第4引数に加算関数をラムダ式に定義する。なお、vectorがクラスでなく単体intやstringの配列なら第4引数(加算関数)の定義は不要であり、自動加算される。
トータルのサンプルソースは以下。
C/C++ | std_accumulate.cpp | GitHub Source |
- #include <iostream>
- #include <vector>
- #include <string>
- #include <numeric>
- class Test {
- public:
- int number;
- std::string string;
- Test(int n, std::string s) {
- number = n;
- string = s;
- }
- };
- int main(void) {
- std::vector<Test> tests = std::vector<Test>();
- tests.push_back(Test(1, std::string("あ")));
- tests.push_back(Test(2, std::string("い")));
- tests.push_back(Test(3, std::string("う")));
- tests.push_back(Test(4, std::string("え")));
- tests.push_back(Test(5, std::string("お")));
- int number_all = std::accumulate( tests.begin(), tests.end(), 0,
- []( int n, auto &c ){ return n + c.number; } );
- std::string string_all = std::accumulate( tests.begin(), tests.end(), std::string(""),
- []( std::string s, auto &c ){ return s + c.string; } );
- std::cout << "number_all=" << number_all << ", string_all=" << string_all << std::endl;
- return 0;
- }
コンパイル、実行結果
- g++ std_accumulate.cpp
- ./a.out
- number_all=15, string_all=あいうえお
Android
iPhone/iPad
Flutter
MacOS
Windows
Debian
Ubuntu
CentOS
FreeBSD
RaspberryPI
HTML/CSS
C/C++
PHP
Java
JavaScript
Node.js
Swift
Python
MatLab
Amazon/AWS
CORESERVER
Google
仮想通貨
LINE
OpenAI/ChatGPT
IBM Watson
Microsoft Azure
Xcode
VMware
MySQL
PostgreSQL
Redis
Groonga
Git/GitHub
Apache
nginx
Postfix
SendGrid
Hackintosh
Hardware
Fate/Grand Order
ウマ娘
将棋
ドラレコ
※本記事は当サイト管理人の個人的な備忘録です。本記事の参照又は付随ソースコード利用後にいかなる損害が発生しても当サイト及び管理人は一切責任を負いません。
※本記事内容の無断転載を禁じます。
※本記事内容の無断転載を禁じます。
【WEBMASTER/管理人】
自営業プログラマーです。お仕事ください!ご連絡は以下アドレスまでお願いします★
【キーワード検索】
【最近の記事】【全部の記事】
ファイアウォール内部のGradio/WebUIを外部からProxyPassを通して使うオープンソースリップシンクエンジンSadTalkerをDebianで動かす
ファイアウォール内部のOpenAPI/FastAPIのdocsを外部からProxyPassで呼ぶ
Debian 12でsshからshutdown -h nowしても電源が切れない場合
【Windows&Mac】アプリのフルスクリーンを解除する方法
Debian 12でtsコマンドが見つからないcommand not found
Debian 12でsyslogやauth.logが出力されない場合
Debian 12で固定IPアドレスを使う設定をする
Debian 12 bookwormでNVIDIA RTX4060Ti-OC16GBを動かす
【Debian】apt updateでCD-ROMがどうのこうの言われエラーになる場合
【人気の記事】【全部の記事】
進研ゼミチャレンジタッチをAndroid端末化する【Windows10】リモートデスクトップ間のコピー&ペーストができなくなった場合の対処法
Windows11+WSL2でUbuntuを使う【2】ブリッジ接続+固定IPの設定
GitLabにHTTPS経由でリポジトリをクローン&読み書きを行う
【C/C++】小数点以下の切り捨て・切り上げ・四捨五入
【Apache】サーバーに同時接続可能なクライアント数を調整する
Windows11のコマンドプロンプトでテキストをコピーする
DebianにウェブサーバーApache2をセットアップ
VirtualBoxの仮想マシンをWindows起動時に自動起動し終了時に自動サスペンドする
Debian 12で固定IPアドレスを使う設定をする
【カテゴリーリンク】
Android
iPhone/iPad
Flutter
MacOS
Windows
Debian
Ubuntu
CentOS
FreeBSD
RaspberryPI
HTML/CSS
C/C++
PHP
Java
JavaScript
Node.js
Swift
Python
MatLab
Amazon/AWS
CORESERVER
Google
仮想通貨
LINE
OpenAI/ChatGPT
IBM Watson
Microsoft Azure
Xcode
VMware
MySQL
PostgreSQL
Redis
Groonga
Git/GitHub
Apache
nginx
Postfix
SendGrid
Hackintosh
Hardware
Fate/Grand Order
ウマ娘
将棋
ドラレコ